In the preceding code block, we created the exerciseList signal by declaring it to contain ExerciseSetList and initializing it with an empty list. Then, we changed the getInitialList method toupdate the exerciseList signal based on the API return. We also changed the delete method to update the signal after deleting the diary entry.
As we are changing the behavior of the function, we also need to exclude the diaryResolver function as now, the service will manage the query in the API and the component will consume the created signal.
In the ListEntriesComponent component, we will refactor to consume the signal list we created:
export class ListEntriesComponent {
@Output() editEvent = new EventEmitter<ExerciseSet>();
@Output() deleteEvent = new EventEmitter<string>();
private exerciseSetsService = inject(ExerciseSetsService);
exerciseList = this.exerciseSetsService.exerciseList;
}
In the preceding code block, we replace the component’s input with the ExerciseSetsService service and receive the exerciseList signal from it.
We will change the ListEntriesComponent component template as follows:
<section class=”mb-8″>
<h2 class=”mb-4 text-xl font-bold”>List of entries</h2>
<ul class=”rounded border shadow”>
@for (item of exerciseList(); track item.id) {
<li>
<app-entry-item
[exercise-set]=”item”
(deleteEvent)=”deleteEvent.emit($event)”
(editEvent)=”editEvent.emit($event)”
/>
</li>
} @empty {
<div>
No Items!
</div>
}
</ul>
</section>
The @for command is prepared to read the content of a signal, including checking the type of value contained in it.
To finish this refactoring, we will change the template of the ‘DiaryComponent’ component:
<app-list-entries
(deleteEvent)=”deleteItem($event)”
(editEvent)=”editEntry($event)”
/>
We removed the exercise list from the app-list-entries component as it will manage the state itself.
After changing the template, we can change the DiaryComponent component:
ngOnInit(): void {
this.exerciseSetsService.getInitialList();
}
deleteItem(id: string) {
this.exerciseSetsService.deleteItem(id);
}
As the state is now managed by the ExerciseSetsService service, we are simplifying the component by just calling the service’s methods, without having to manage subscriptions to observables.
With the state managed by Signals, we can add a new feature here on this screen. Let’s assume that we need to inform the total training volume in the diary, that is, the total amount of exercise performed.
To have this information and react to events such as the deletion or inclusion of an entry, we can use Angular Signals!
In the DiaryComponent component, we will make the following change:
volume = computed<number>(() =>
this.exerciseSetsService
.exerciseList()
.reduce(
(volume, exerciseSet) => volume + exerciseSet.reps * exerciseSet.sets,
0
)
);
We create a new computed signal called ‘volume’ and perform the calculation in it based on the value contained in the ‘exerciseList’ signal.
To use this new signal, let’s change the template:
<header class=”bg-blue-500 py-4 text-white”>
<div class=”mx-auto max-w-6xl px-4″>
<h1 class=”text-2xl font-bold”>Workout diary – Total Volume: {{volume()}} </h1>
</div>
</header>
We are consuming the volume signal by calling the signal directly in the template. By running our project, we can notice that this volume signal reacts to the changes we make in our list of exercises.
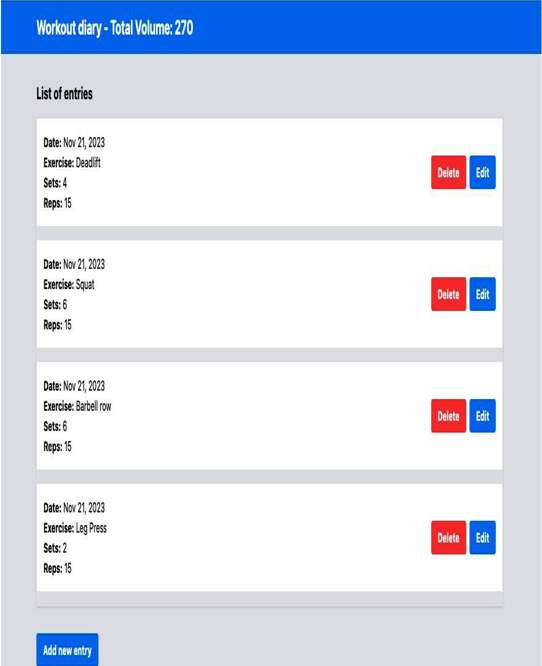
Figure 12.2 – Lazy-loaded bundle
Signals are elements that will be increasingly improved by the Angular team, giving more control over the reactivity of our applications. An important point that we need to pay attention to is that Signals will not replace RxJS; in fact, they complement each other as we still need observables to control asynchronous flows and more complex flows, as we studied in Chapter 9, Exploring Reactivity with RxJS.
Summary
In this chapter, we explored the possibilities that the future of the Angular framework can offer us. We learned how to update our project to new versions of Angular, an ongoing activity as the framework continues to evolve. We understood how Angular versioning works and the importance of continually updating our project, from the point of view of security, performance, and new features. Then, we changed our application to use the new template expressions, which, in addition to simplifying, can, depending on the case, improve the performance of our applications. With this improvement in template expressions, we looked at the defer expression, which allows for the lazy loading of components within templates, giving us new options for optimizing interfaces with complex components. We also learned how to use the View Transactions API to improve our users’ experience with animations between page changes. Finally, we explored Angular Signals and simplified the state management of our application with this new element that complements RxJs. Angular is a framework that never stops evolving, as our users never stop demanding new features. In this chapter, we learned how to stay up to date with Angular.
No Responses